Tazids: A Hands-On Machine Learning Library for Beginners 🚀
Scikit-learn? Nah, It’s Tazids!
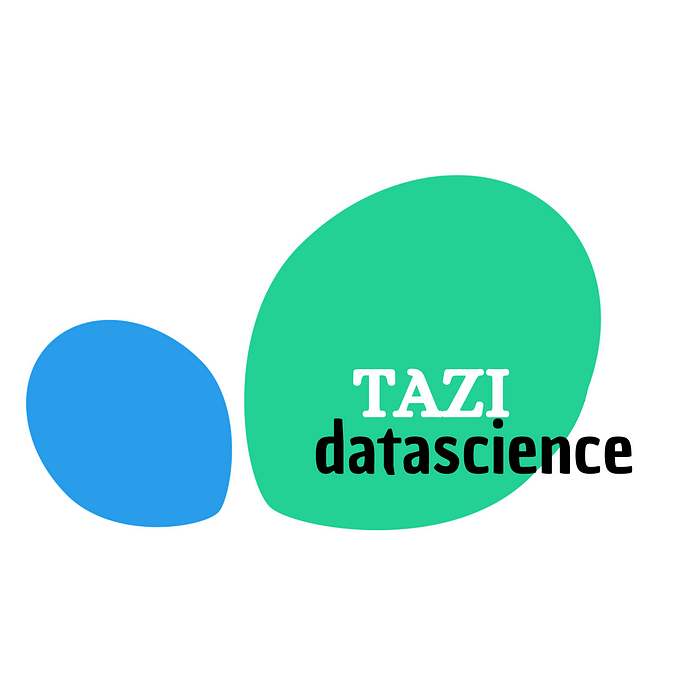
Just kidding — Tazids isn’t here to compete with Scikit-learn… but it might be better in one way.
Let me explain.
As a data scientist, I’ve been a huge consumer of machine learning libraries like Scikit-learn, TensorFlow, and PyTorch — and for good reason. They’re fast, easy to use, and abstract away the complex math so you can focus on solving problems. But this year, I found myself asking a big question:
“Could I build these algorithms from scratch?”
Machine learning models, at their core, are just math. So why not dive deeper and code them myself? Armed with countless resources online, I set out to understand the inner workings of the algorithms I’ve used so often. That’s how Tazids was born.
It started with Linear Regression — implementing it from scratch wasn’t as hard as I expected. I broke it down step by step, built the algorithm in Python, and realized how satisfying (and enlightening!) it was to see the math come to life in code.
Now, I’m introducing Tazids to the world, hoping it helps others learn machine learning the same way it helped me.
What Is Tazids?
Tazids is an educational Machine Learning library designed to help learners get hands-on with foundational algorithms. Unlike complex, production-grade libraries like Scikit-learn, Tazids keeps things simple and transparent.
With Tazids, you don’t just use a pre-built model — you understand how it works under the hood. You can tweak parameters, modify the code, and see how your changes impact the algorithm’s performance.
Here’s what makes Tazids unique:
- Straightforward Code: The implementations are clean, simple, and easy to follow.
- Hands-On Learning: It’s built for experimentation. Change a line of code, adjust the learning rate, or add a new feature — you’ll learn by doing.
- Educational Focus: Tazids isn’t about speed or scalability. It’s about learning the concepts and math behind machine learning.
What’s Inside?
Tazids includes implementations of several key machine learning algorithms, each designed to be as educational as possible. Here’s a quick look:
Linear Regression
The foundation of machine learning, Linear Regression predicts a continuous target variable based on input features. In Tazids, I implemented it using gradient descent, so you can see how the model minimizes error step by step.
from tazids.regressor import LinearRegression
import numpy as np
# Generate synthetic data
np.random.seed(42)
X = np.random.rand(100, 1) * 10
y = np.random.rand(100) * 100
# Train the model
model = LinearRegression()
model.fit(X, y, learning_rate=0.001, iters=1000)
# Make predictions
predictions = model.predict(X)


KMeans Clustering
Unsupervised learning? Tazids has you covered with KMeans, an algorithm that groups data points into clusters. You’ll see exactly how centroids are initialized, updated, and optimized.
from tazids.cluster import KMeans
import numpy as np
import matplotlib.pyplot as plt
# Generate synthetic data
np.random.seed(42)
X = np.random.rand(100, 2) * 10
# Fit KMeans model
model = KMeans()
model.fit(X, k=3)
# Visualize clusters
plt.scatter(X[:, 0], X[:, 1], c=model.labels, cmap='viridis')
plt.scatter(model.centroids[:, 0], model.centroids[:, 1], c='red', marker='x', s=200)
plt.show()

Decision Tree
Ever wonder how decision trees split data into branches? Tazids includes a simple implementation that shows you how features are selected and splits are calculated.
from tazids.tree import DecisionTree
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
# Import Dataset
data = load_iris()
X = data.data
y = data.target
# Fit Tree model
model = DecisionTree()
model.fit(X,y)
# Visualize results
model.predict(X)

Why I Built Tazids
Let’s be real: Tazids isn’t here to replace Scikit-learn (at least not yet 😅). But building it was an incredible experience for me as a data scientist. It forced me to understand the math, the logic, and the mechanics behind the models I’ve used for years.
If you’re like me — someone who wants to dig deeper and truly understand machine learning — Tazids is for you. It’s a tool to level up your knowledge and appreciate what’s happening behind the scenes.
Get Started
Ready to try Tazids? It’s easy:
pip install tazids
Once installed, you can start experimenting with the library and learning how machine learning models work from the ground up.
In the world of machine learning, simplicity is underrated. While production-grade libraries are amazing, there’s something special about building models from scratch.
Tazids was born out of curiosity, and I hope it sparks the same curiosity in you. Whether you’re a beginner or a seasoned data scientist, Tazids can help you see machine learning in a new light.
So, what are you waiting for? Dive in, explore the code, and discover the joy of understanding machine learning — one algorithm at a time.
You can find Tazids on PyPI and GitHub. Happy learning!
What algorithm would you like to see in Tazids next?
About Me
Hi, I’m Mohannad Tazi, a data scientist driven by the mission to make machine learning accessible, transparent, and enjoyable for everyone. I created Tazids to empower learners to dive deep into the foundations of machine learning and truly grasp the magic behind the algorithms.
💡 Let’s connect!
- LinkedIn: Let’s exchange ideas, collaborate, or chat about all things ML : mohannad-tazi
- Website: Explore my projects, including generative AI, IoT, and more, at
Feel free to reach out — I’m always excited to discuss new ideas, projects, and collaborations!