Mastering Loss Functions: From Fundamentals to Designing Your Own
In machine learning and deep learning, the choice of a loss function is critical. It is the cornerstone that defines how a model “learns”, evaluates its predictions, and improves through optimization. Beyond standard loss functions, we will explore the nuances of creating custom loss functions, their mathematical foundations, and practical design considerations.
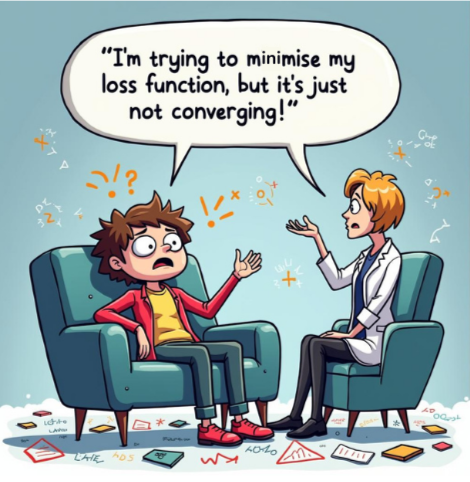
1. What Are Loss Functions?
At its core, a loss function quantifies the difference between a model’s predictions (y^) and the true labels (y). This quantification, often called the “error,” is minimized during training to improve the model’s predictions. Mathematically, a loss function L maps predicted and actual values to a scalar value:
Loss functions can broadly be categorized into pointwise loss functions, applied to individual data points (e.g., Mean Squared Error), and distributional loss functions, considering distributions (e.g., Kullback-Leibler Divergence).
2. Common Loss Functions: Intuition and Mathematics
2.1. For Regression
Mean Squared Error (MSE):
- Use case: When all errors are equally important.
- Behavior: Penalizes large errors more due to the squaring.
Mean Absolute Error (MAE):
- Use case: When robustness to outliers is needed.
- Behavior: Linear growth for large errors.
Huber Loss:
Combines MSE and MAE for robustness:
- Use case: Balances sensitivity to small and large errors.
2.2. For Classification
Cross-Entropy Loss:
For binary classification:
For multi-class classification:
- Use case: Probabilistic classification tasks.
- Behavior: Heavily penalizes wrong confident predictions.
Hinge Loss:
Used in SVMs:
- Use case: Tasks requiring margin maximization.
- Behavior: Encourages separation with a margin.

3. Beyond Standard Loss Functions: Why Customize?
Standard loss functions often assume generic objectives, but real-world problems are rarely generic. Custom loss functions allow for domain-specific tuning, such as:
- Penalizing false positives more than false negatives (e.g., fraud detection).
- Prioritizing certain classes in imbalanced datasets.
- Incorporating domain knowledge into optimization.
Example: Asymmetric Loss
Asymmetric losses penalize overestimation and underestimation differently. For instance, in predicting house prices:
4. How to Design a Loss Function
4.1. Characteristics of a Good Loss Function
- Differentiability: Necessary for optimization algorithms like gradient descent.
- Well-Defined Gradients: Gradients should not explode or vanish.
- Alignment with Objectives: Should reflect the practical goals of the task.
- Robustness: Handle outliers and noisy data effectively.
4.2. Step-by-Step Guide
- Define the Problem Objective: Clearly state the trade-offs you want the model to optimize.
- Design the Mathematical Formulation: Ensure the function reflects the priorities (e.g., penalize large positive errors).
- Implement in Code: Example in PyTorch:
import torch
class CustomLoss(torch.nn.Module):
def __init__(self, alpha, beta):
super(CustomLoss, self).__init__()
self.alpha = alpha
self.beta = beta
def forward(self, y_true, y_pred):
diff = y_pred - y_true
loss = torch.where(diff < 0, self.alpha * diff**2, self.beta * diff**2)
return torch.mean(loss)
4. Test and Validate: Compare the performance of your loss function against standard ones.
5. Case Study: ASLAN Loss
The ASLAN loss was introduced to address imbalanced datasets with specific focus on optimizing rare classes. It dynamically adjusts the penalty for each class based on its frequency:
Key Benefits:
- Encourages learning rare classes.
- Combines principles of class weighting and robust margins.
6. Can We Create the “Perfect” Loss Function?
While no loss function is universally optimal, a tailored loss function aligns the model closer to the problem’s requirements. Some areas to innovate include:
- Adaptive Loss Functions: Dynamically adjust penalties based on epoch or dataset properties.
- Hybrid Loss Functions: Combine multiple objectives (e.g., Cross-Entropy + Dice Loss in image segmentation).
- Task-Specific Loss Functions: Incorporate domain-specific metrics directly into the loss (e.g., BLEU score in NLP tasks).
7. Conclusion
Loss functions are more than mathematical expressions; they embody the objectives of a learning system. By understanding their behavior and tailoring them to your needs, you can unlock new possibilities in machine learning and deep learning.
Have you ever created a custom loss function? Share your thoughts and challenges, feel free to connect with me on my journey through Data Science, AI, and beyond!
👉 LinkedIn: mohannad-tazi
Check out more updates and projects on my website: